Control cPanel email autoresponder with a Google Calendar
You know how limited the cPanel email autoresponder is - you can only program in one response, the next one - and if you want to have different responses for different days you’ve got to program in each one every time…
Not any more! With my little script you can set up a Google Calendar to program the autoresponder for you. You simply create an event for whenever you want the autoresponder on. The event’s name and description tell the autoresponder what to say. You can use repeating events, day-long events, or short one hour events if you want to.
Here’s what an autoresponder calendar looks like:
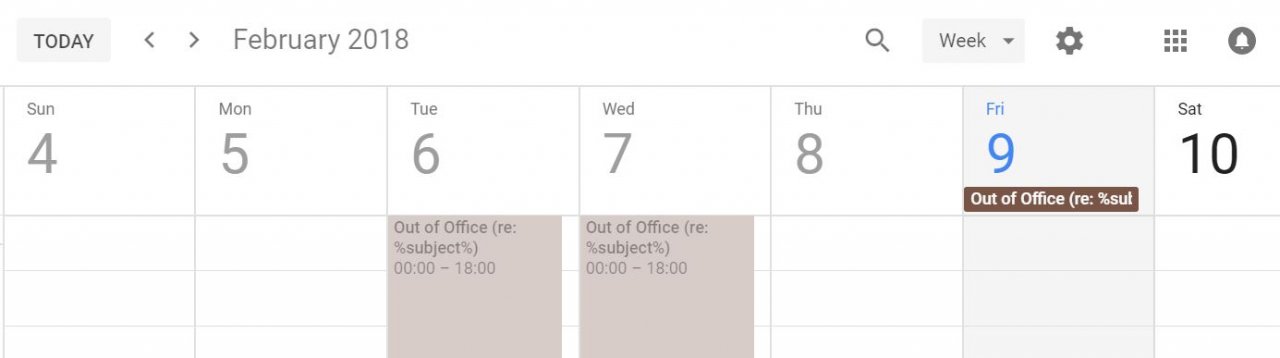
And here’s a sample “event”
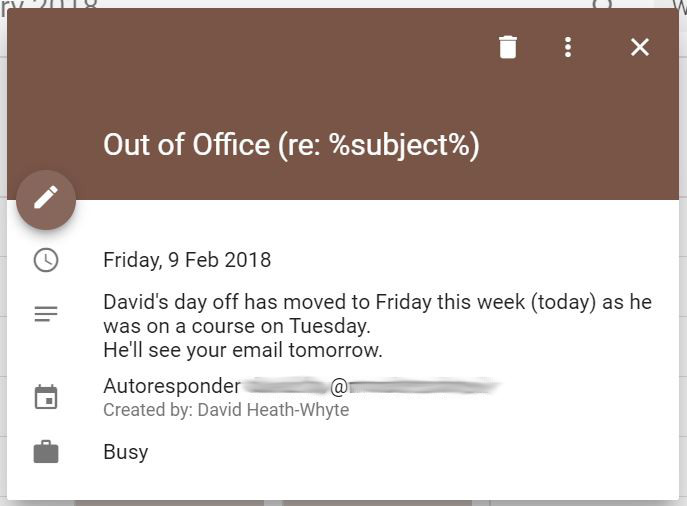
Script and Installation
<?php
// autoresponderCal.php
// uses a .ics calendar to set the autoresponder
// Subject line of the Calendar event becomes email subject
// Description of Calendar event becomes email content
// the email address
define ('AUTORESP_EMAIL', 'your.email@example.com');
your.
// the location of the calendar file
// "secret address in ical format" from Google Calendar settings
define ('AUTORESP_CALFILE', 'https://calendar.google.com/calendar/ical/whatever-the-code-is-for-your-calendar/basic.ics');
// the location of cpanel autoresponder files
define ('AUTORESP_FILES', '/home/your-cpanel-account/.autorespond/');
// ##################################################################
// load dependencies
require_once dirname(__FILE__) . '/Carbon/Carbon.php';
require_once dirname(__FILE__) . '/ICal/ICal.php';
require_once dirname(__FILE__) . '/ICal/Event.php';
use Ical\Ical;
// get the calendar file
try {
$ical = new ICal(false, array(
'defaultSpan' => 2, // Default value
'defaultTimeZone' => 'UTC',
'defaultWeekStart' => 'MO', // Default value
'disableCharacterReplacement' => false, // Default value
'skipRecurrence' => false, // Default value
'useTimeZoneWithRRules' => false, // Default value
));
$ical->initUrl(AUTORESP_CALFILE);
} catch (\Exception $e) {
die($e);
}
// get one week of events
$events = $ical->eventsFromInterval('1 week');
if ($events) {
// get the first event
$event = $events[0];
// echo $event->printData();
// get the email address from the location - NOT FOR NOW
// $email = ($event->location == '') ? AUTORESP_EMAIL : $event->location;
$email = AUTORESP_EMAIL;
// we want start and end times as timestamp
// make an array
$autoR = array(
'start' => strtotime($event->dtstart),
'stop' => strtotime($event->dtend),
'interval' => 43200
);
// and encode it
$json = json_encode($autoR);
// get the subject line from the calendar event
$subject = $event->summary;
// get the email content from the description
$content = $event->description;
// create a text file in the correct format for the autoresponder:
$text = 'From: "%from%" <' . $email . '>' . "\n"
. "Content-type: text/plain; charset=utf-8\n"
. "Subject: " . htmlspecialchars_decode($subject) . "\n"
. "\n"
. htmlspecialchars_decode($content);
// now save the text file and the json info in the right place
file_put_contents(AUTORESP_FILES . $email . '.json', $json);
file_put_contents(AUTORESP_FILES . $email, $text);
// echo "<br>$json<br>$text";
}
?>
Folders
I’ve installed this into a new folder “autoresponderCal” in the root of my cpanel’s home folder on the server. In this new folder you also need “Carbon” and “ICal” folders.
The Carbon folder has Carbon.php from here: https://github.com/briannesbitt/Carbon/blob/master/src/Carbon/Carbon.php
The ICal folder contains two files ICal.php and Event.php from this helpful ics-parser: https://github.com/u01jmg3/ics-parser/tree/master/src/ICal
So here’s the folder structure:
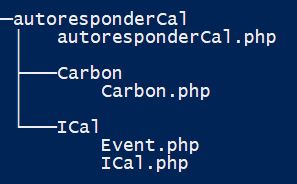
Script changes
There are some lines you need to change in the autoresponderCal.php script:
// the email address
define ('AUTORESP_EMAIL', 'your.email@example.com');
your.
// the location of the calendar file
// "secret address in ical format" from Google Calendar settings
define ('AUTORESP_CALFILE', 'https://calendar.google.com/calendar/ical/whatever-the-code-is-for-your-calendar/basic.ics');
// the location of cpanel autoresponder files
define ('AUTORESP_FILES', '/home/your-cpanel-account/.autorespond/');
Cron job
Yes, you need a cron job to run the script and set the autoresponder.
Here’s what I set up:

That’s via cPanel, it’s an hourly cron job. That way I can setup autoresponder calendar events that change on the hour.
Google Calendar
You need to set up a new calendar that is specifically for autoresponderCal.
Once you’ve done that, you need the “secret address in ical format” from the calendar’s settings page, put that in the script (see above).
You can then make a new event: the name of the event becomes your email subject line, and the description becomes the email text, including whatever html you use (bold, italic, new lines etc).
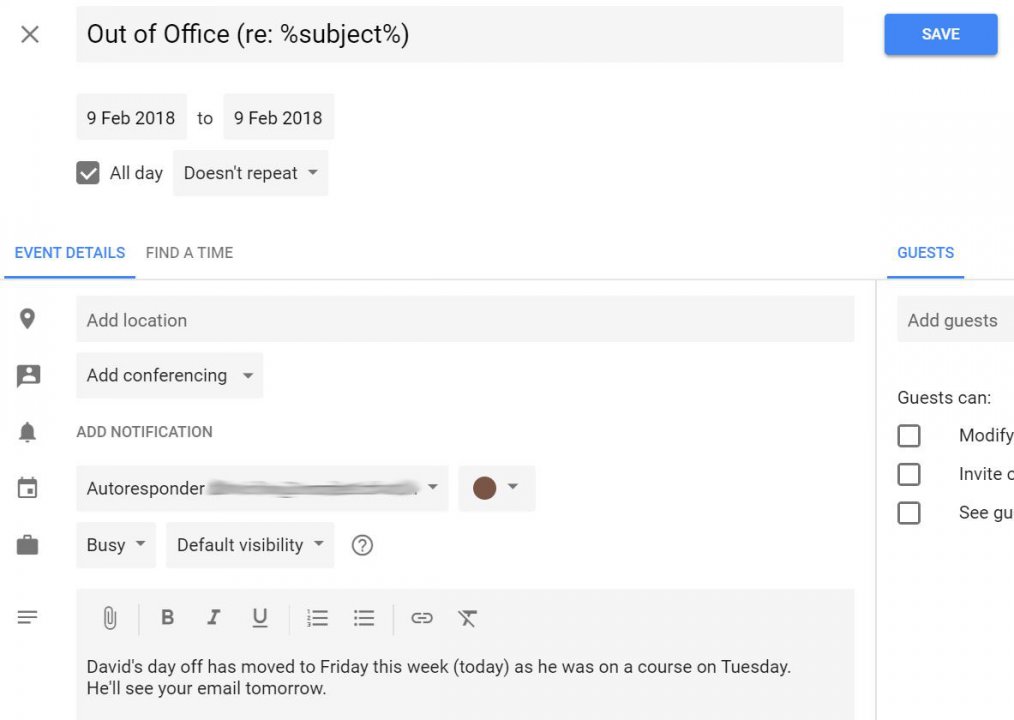
And that’s it. I think - of course I’ll update and edit this if more help is needed! Let me know how you get on.
NB: Security
You use this script at your own risk. It may have security flaws that I am not aware of.
Make sure your calendar is private - there are probably scary things that could happen if you let someone else near it.